List/Grid View
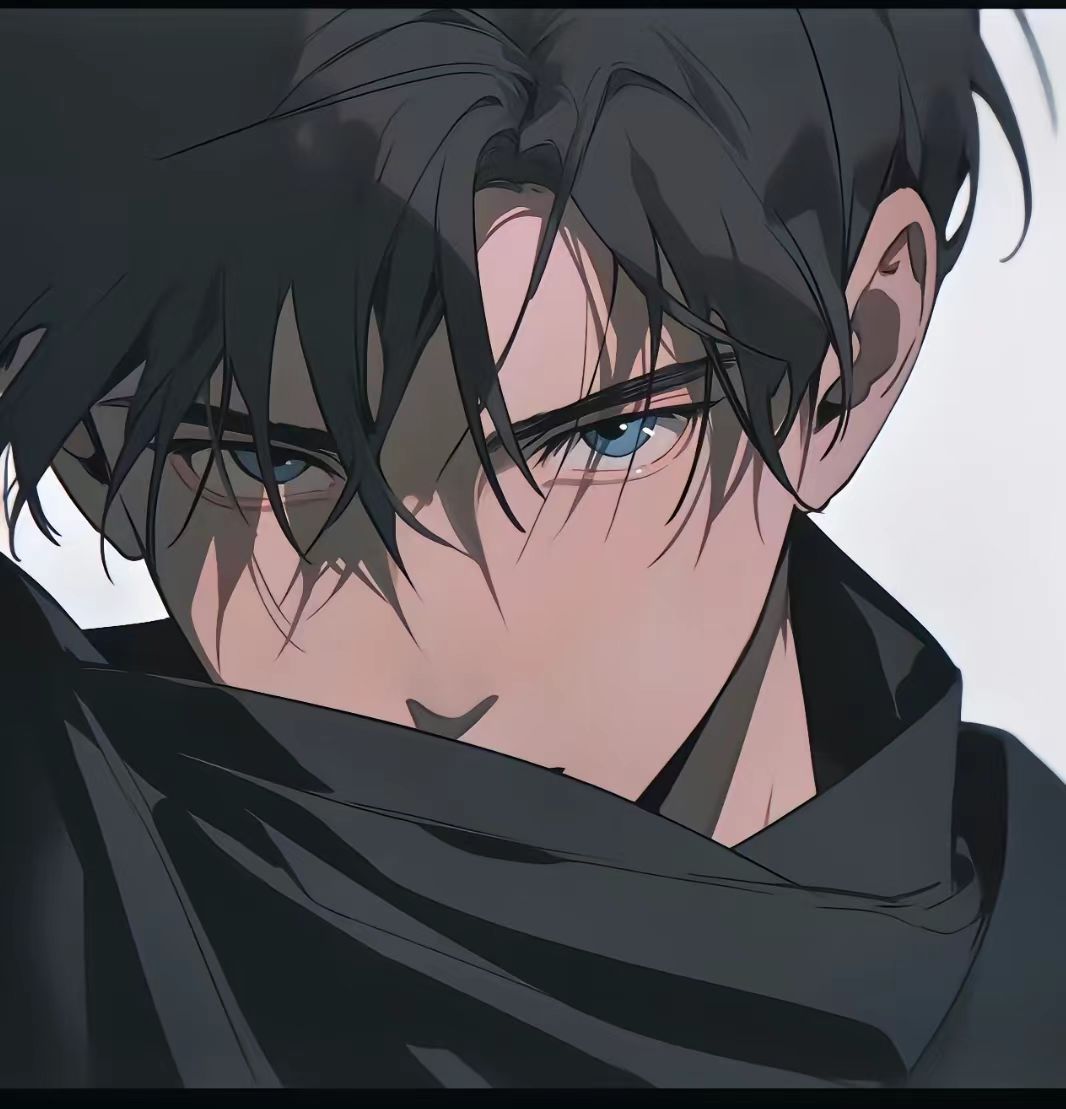
网格可滑动表格
摘要 (GridView)
在网格中使用 widget
当列的内容超出渲染容器的时候,它会自动支持滚动。
GridView.count 允许你制定列的数量
GridView.extent 允许你制定单元格的最大宽度
使用 GridView.count 创建一个网格,它在竖屏模式下有两行,在横屏模式下有三行。
可以通过为每个 GridTile 设置 footer 属性来创建标题。
https://github.com/cfug/flutter.cn/tree/main/examples/layout/gallery/lib/grid_list_demo.dart
1 | Widget _buildGrid() => GridView.extent( |
ListView
ListView,一个和列很相似的 widget,当内容长于自己的渲染盒时,就会自动支持滚动。
标准的 ListView 构造函数适用于短列表,对于具有大量列表项的长列表,需要用 ListView.builder 构造函数来创建。
与标准的 ListView 构造函数需要一次性创建所有列表项不同的是, ListView.builder 构造函数只在列表项从屏幕外滑入屏幕时才去创建列表项。
摘要 (ListView)
一个用来组织盒子中列表的专用 Column
可以水平或者垂直布局
当监测到空间不足时,会提供滚动
比 Column 的配置少,使用更容易,并且支持滚动
通过指定 scrollDirection 的值为水平方向,来覆盖默认的竖直方向
1 | Widget _buildList() { |
下面举例创建一个带数据的列表
1. 创建数据源
首先,需要获取列表的数据源。例如,数据源可以是消息集、搜索结果集或者商店商品集。大部分情况下,这些数据来自于网络请求或者数据库获取。
在下面的例子,使用 List.generate 构造函数生成包含 10,000 个字符串的集合。
1 | List<String>.generate(10000, (i) => 'Item $i'), |
2. 将数据源渲染成组件
为了将字符串集合展示出来,需要通过 ListView.builder 把集合中的每个字符串都渲染成组件。
在下面的例子中,将会把每个字符串用单行列表项显示在列表中。
1 | ListView.builder( |
完整代码
1 | import 'package:flutter/material.dart'; |
创建展示不同类型内容的列表
比方说,我们可能在开发一个列表,它显示一个标题,后跟一些与标题相关的项目,然后是另一个标题,依此类推。
创建一个拥有不同类型项目的数据源
将数据源的数据转换成列表 widget
- 创建一个具有不同类型项目的数据源
为了表示 List 中不同类型的项,我们需要为每一个类型的项目定义一个类。
在这个例子中,我们将制作一个展示了标题,后面有五条消息的应用。因此,我们将创建三个类:ListItem、HeadingItem 和 MessageItem。
你可以通过以下步骤,用 Flutter 创建这样的结构:
1 | /// The base class for the different types of items the list can contain. |
1 | final items = List<ListItem>.generate( |
- 将数据源的数据转换成列表 widget
为了把每一个项目转换成 widget,我们将采用 ListView.builder() 构造方法。
通常,我们需要提供一个 builder 函数来确定我们正在处理的项目类型,并返回该类型项目的相应 widget。
1 | ListView.builder( |
- Title: List/Grid View
- Author: 人间烟火/张佳伟版
- Created at: 2024-04-21 21:56:03
- Updated at: 2024-04-21 21:28:37
- Link: https://945912035.github.io/2024/04/21/2024-4-21/
- License: This work is licensed under CC BY-NC-SA 4.0.